The new blog address is: http://escogitare.com
Escogitare
Thursday, June 7, 2012
Monday, October 3, 2011
Icons on iOS and OS X
Sometime I need some small table to resume quickly the artwork required for the iOS and OsX applications. They are often the same image, but in different resolutions. Here they are:
More information for Os X here:
Icon Design Guidelines
More information for iOS here: Custom Icon and Image Creation Guidelines
OsX
Resolution | Notes | |
---|---|---|
Icons | 1024 x 1024 pixels 512 x 512 pixels 256 x 256 pixels 128 x 128 pixels 32 x 32 pixels 16 x 16 pixels | |
Toolbar icons (inside control) | 19 x 19 pixels | Outline style, recommanded to be black |
Toolbar icon buttons | 32 x 32 pixels | Color with antialiasing |
Sidebar icons | 16 x 16 pixels 18 x 18 pixels 32 x 32 pixels | Black and white with alpha, all three resolutions required |
iOS
Resolution | Notes | |
---|---|---|
Icon for iPhone | 57 x 57 pixels | Suggested name: Icon.png. No shadow or round corners required. |
Icon for iPhone with Retina Display | 114 x 114 pixels | Suggested name: Icon@2x.png. No shadow or round corners required. |
Icon for iPad | 72 x 72 pixels | Suggested name: Icon-72.png. No shadow or round corners required. |
Small icons iPhone (Spotlight and settings) | 29 x 29 pixels | |
Small icons iPhone High Resolution (Spotlight and settings) | 58 x 58 pixels | |
Small icons iPad (Spotlight) | 50 x 50 pixels | It trims the side pixels (safe area 48x48) |
Small icons iPhone (settings) | 29 x 29 pixels | |
Toolbar icons for iPhone | 20 x 20 pixels | Approximately |
Toolbar icons for iPhone High Resolution | 40 x 40 pixels | Approximately |
Toolbar icons iPad | 20 x 20 pixels | Approximately |
Tab bar icons iPhone | 30 x 30 pixels | Approximately |
Tab bar icons iPhone High resolution | 60 x 60 pixels | Approximately |
Tab bar icons iPad | 30 x 30 pixels | Approximately |
Open source/free toolbar icon sets
Some link to free/open source iPhone toolbar icon set:Tuesday, September 27, 2011
How to take a screenshot with the Android SDK
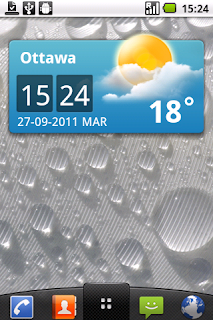
So, go to that directory and start it:
cd [sdkdir]/tools
./ddms
Then go on the Device menu ant click on Screen capture. Then save the image file.
If you want to take a screenshot from Eclipse, it is even simpler.
Just set the DDMS perspective:
Look for the camera button, and click on it to show the following menu:
Here you can find the "Screen Capture" command.
Monday, September 19, 2011
How to check if our app is running on an iPad or iPhon
The easiest way is using the
In the same way:
The macro uses the
UI_USER_INTERFACE_IDIOM
macro. Only SDKs 3.2 an later support it. Here an example:if (UI_USER_INTERFACE_IDIOM()==UIUserInterfaceIdiomPad)
{
NSLog(@"I'm on iPad");
}
In the same way:
if (UI_USER_INTERFACE_IDIOM()==UIUserInterfaceIdiomPhone) {
NSLog(@"I'm on iPhone or on an iPod touch");
}
The macro uses the
[[UIDevice currentDevice] userInterfaceIdiom]
method. If you are sure that your SDK supports the userInterfaceIdiom selector, you can also use something like this:[[UIDevice currentDevice] userInterfaceIdiom]==UIUserInterfaceIdiomPad
Thursday, September 8, 2011
Android's services
Android uses services to perform long running jobs, or background tasks (think to a network interaction or an mp3 player). How can we start a service?
We should begin extending the android.app.Service class:
@Override
We should begin extending the android.app.Service class:
public class TestService extends Service {
@Override
public IBinder
onBind(Intent arg0) {
return null;
}
public void onCreate () {
Log.i("onCreate", "onCreate");
}
public int
onStartCommand(Intent intent, int flags, int startId) {
Log.i("onStartCommand", "onStartCommand");
Toast.makeText(this, "Hi, this is a service!",
Toast.LENGTH_LONG).show();
return START_STICKY;
}
}
The two most important methods, for the purposes
of this post, are onCreate and onStartCommand. Android invokes the first, when
the service is created, and the second one, when it is executed. The
Toast.makeText(…) command is a nice way to give notifications to the users.
Please note that the onStartCommand
callback exists only in the versions 2.0 and later. In older versions you should
override the onStart method (now deprecated).
Once you have you service’s skeleton, you
can register with the operating system, adding a line to the manifest:
<service android:name="TestService"
android:enabled="true" >service>
Or in a easier way, using the Eclipse interface:
At the end is time to start our service,
using two instructions:
Intent intent = new Intent(this, TestService.class);
startService(intent);
We create an android.content.Intent instance, and then we use
it in the startService method. The Intent, ask you for a context (your calling
Activity is fine), and the implementation class. He will take care of the
instantiation. The startService will call your onStartCommand procedure, passing
it also the intent instance. A nice place to do it could be in the onClick
method:
public void onClick(View v) {
switch (v.getId()) {
case R.id.buttonStart:
Intent
intent = new Intent(this, TestService.class);
startService(intent);
break;
}
}
Friday, September 2, 2011
How to take screenshots directly on the iPhones
If you want to take a screen-shot directly from your iPhone/iPod touch/iPad, and find it directly in your photo library, there is a simple procedure:
The (so called) Home and Sleep buttons are marked in red in the picture.
- Press the Home button, without releasing it;
- Press and release the Sleep button;
- Release the Home button.
The (so called) Home and Sleep buttons are marked in red in the picture.
Wednesday, April 20, 2011
Load an XML file in Mac Os X using Cocoa: NSXMLDocument
Here another snippet on how to load an XML file using the NSXMLDocument class. Our goal is to show an “Open File” dialog box (as said in the last post), chose an xml file, and put it in a data structure (NSXMLDocument)
Here’s the code:
The only “strange” thing suggested by the Apple’s example and reported here, is the double initialization of NSXMLDocument, with different parameters: the first one with NSXMLNodePreserveWhitespace|NSXMLNodePreserveCDATA, the second one with NSXMLDocumentTidyXML.
We are trying to recover from parsing errors. NSXMLDocumentTidyXML reformats the document before the parsing. Hopefully it can correct some error, but it modifies the original file (take a look at the documentation).
More information is in the “Introduction to Tree-Based XML Programming Guide for Cocoa” on the Apple Devveloper web site.
Here’s the code:
-(BOOL) LoadXml : (NSError **)err { NSURL *fileUrl = nil; NSOpenPanel* openDlg = [NSOpenPanel openPanel]; [openDlg setCanChooseFiles:YES]; [openDlg setCanChooseDirectories:NO]; [openDlg setAllowsMultipleSelection: NO]; [openDlg setAllowedFileTypes:[NSArray arrayWithObjects:@"xml", nil]]; if ( [openDlg runModal] == NSOKButton ) { fileUrl = [openDlg URL]; } if (fileUrl==nil) { return NO; } xmlDoc = [[NSXMLDocument alloc] initWithContentsOfURL:fileUrl options:(NSXMLNodePreserveWhitespace|NSXMLNodePreserveCDATA) error:err]; if (xmlDoc == nil) { xmlDoc = [[NSXMLDocument alloc] initWithContentsOfURL:fileUrl options:NSXMLDocumentTidyXML error:&err]; } if (xmlDoc == nil) return NO; ... }
The only “strange” thing suggested by the Apple’s example and reported here, is the double initialization of NSXMLDocument, with different parameters: the first one with NSXMLNodePreserveWhitespace|NSXMLNodePreserveCDATA, the second one with NSXMLDocumentTidyXML.
We are trying to recover from parsing errors. NSXMLDocumentTidyXML reformats the document before the parsing. Hopefully it can correct some error, but it modifies the original file (take a look at the documentation).
More information is in the “Introduction to Tree-Based XML Programming Guide for Cocoa” on the Apple Devveloper web site.
Subscribe to:
Posts (Atom)